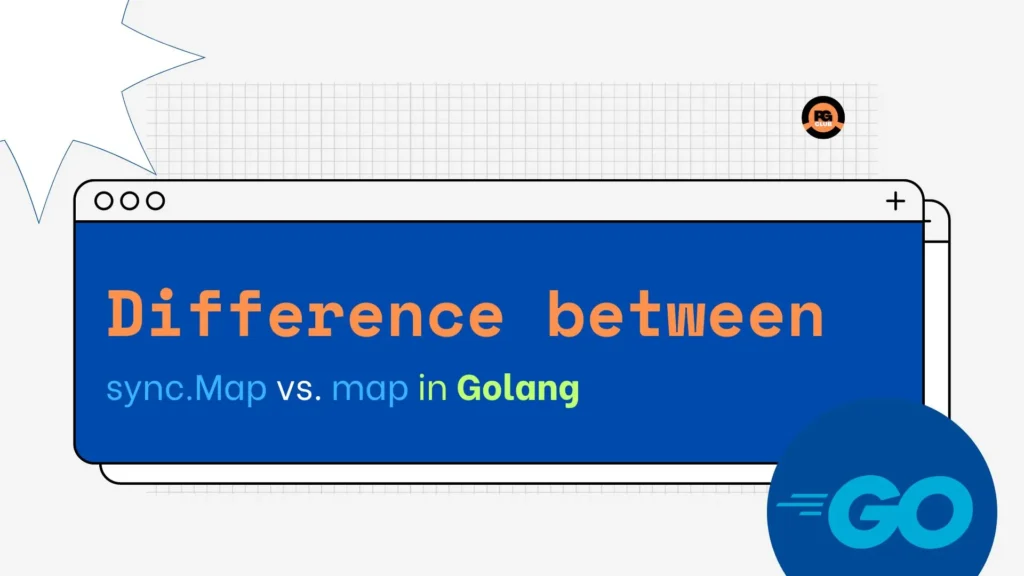
In Go, maps are commonly used for storing key-value pairs. However, when it comes to concurrent programming, we need to be careful about accessing shared data to avoid race conditions. To solve this problem, Go provides the sync.Map type, which is a safe and concurrent alternative to maps. In this article, we’ll discuss the differences between sync.Map and maps in Go, and how to use them in different scenarios.
Maps in Go
A map is a collection of unordered key-value pairs, where the keys are unique and the values can be of any type. Maps are commonly used for storing data in Go, and they can be created using the make function, Below is the following code:
m := make(map[string]int)
In this example, we created an empty map that can store integer values with string keys. We can add or update values in the map using the square bracket notation, as mentioned in the following code:
m["foo"] = 1 m["bar"] = 2
We can also retrieve values from the map using the square bracket notation, as mentioned in the following code:
fmt.Println(m["foo"]) // 1
However, maps are not safe for concurrent use. If multiple goroutines try to access or modify a map at the same time, it can cause race conditions and data corruption. To avoid this problem, we need to use a mutex or another synchronization mechanism to ensure that only one goroutine can access the map at a time.
Sync.Map in Go
The sync.Map type is a built-in type in Go that provides a safe and concurrent alternative to maps and it was introduced in go version 1.9. The sync.Map type uses a different internal implementation than maps, which makes it safe for concurrent use without requiring any additional synchronization.
Creating a sync.Map is similar to creating a map, except that we don’t need to use the make function:
var m sync.Map
In this example, we created an empty sync.Map object. We can add or update values in the map using the Store method:
m.Store("foo", 1) m.Store("bar", 2)
We can retrieve values from the map using the Load method:
if v, ok := m.Load("foo"); ok { fmt.Println(v) // 1 }
The Load method returns the value associated with the key if it exists, and a boolean value indicating whether the key exists in the map. We can also delete values from the map using the Delete method:
m.Delete("foo")
The sync.Map type also provides a few other methods, such as Range, which allows us to iterate over all key-value pairs in the map. The Range method takes a function as an argument, which is called for each key-value pair in the map:
m.Range(func(key, value interface{}) bool { fmt.Printf("%v: %v\n", key, value) return true // continue iterating })
The Range function returns true to continue iterating, or false to stop iterating.
Differences between sync.Map and maps
There are several differences between sync.Map and maps in Go as mentioned in below:
- Safety: sync.Map is safe for concurrent use without requiring any additional synchronization, while maps are not safe and require a mutex or another synchronization mechanism to avoid race conditions.
- Initialization: Maps need to be initialized using the make function, while sync.Map does not require initialization.
- Type: Maps are statically typed, while sync.Map is a dynamic type that can store values of different types without needing to specify the types in advance.
- Performance: Maps are faster than sync.Map for non-concurrent use because they have less overhead. However, when multiple goroutines are accessing the same data, sync.Map can be faster because it eliminates the need for locking and unlocking a mutex.
- Copying:Maps are copied by value, while sync.Map is copied by reference. This means that if we pass a map to a function, the function gets a copy of the map, while if we pass a sync.Map, the function gets a reference to the original map.
When to use sync.Map
sync.Map should be used when we need to share data between multiple goroutines and we don’t want to use a mutex or another synchronization mechanism. sync.Map is useful for read-heavy workloads, where multiple goroutines are reading the same data frequently but only a few goroutines are updating the data.
On the other hand, if we have a write-heavy workload, where multiple goroutines are updating the data frequently, we may need to use a mutex or another synchronization mechanism to ensure that only one goroutine can access the data at a time. In this case, using a map with a mutex may be a better option.
Let’s take a look at some examples of how to use sync.Map.
Example 1: Caching
One common use case for sync.Map is caching. In this example, we’ll create a simple HTTP server that returns the current time in ISO 8601 format. We’ll use sync.Map to cache the response for each request, so that we don’t need to generate a new response for every request.
package main import ( "fmt" "log" "net/http" "sync" "time" ) var cache sync.Map func main() { http.HandleFunc("/", handler) log.Println(http.ListenAndServe(":8000", nil)) } func handler(w http.ResponseWriter, r *http.Request) { v, ok := cache.Load(r.URL.Path) if ok { fmt.Fprintln(w, v) return } response := time.Now().UTC().Format(time.RFC3339) cache.Store(r.URL.Path, response) fmt.Fprintln(w, response) }
In this example, we create a sync.Map called cache to store the response for each request. In the handler function, we first check if the response for the current request is already in the cache using the Load method. If it is, we write the response to the client and return early. Otherwise, we generate a new response using time.Now().UTC().Format(time.RFC3339), store it in the cache using the Store method, and write it to the client.
Example 2: Memoization
Another common use case for sync.Map is memoization, which is a technique for caching the results of a function to avoid recomputing them for the same input. In this example, we’ll create a simple function that computes the nth Fibonacci number, and use sync.Map to memoize the results for better performance.
package main import ( "fmt" "sync" ) var memo sync.Map func main() { fmt.Println(fib(10)) fmt.Println(fib(20)) } func fib(n int) int { v, ok := memo.Load(n) if ok { return v.(int) } if n < 2 { memo.Store(n, n) return n } result := fib(n-1) + fib(n-2) memo.Store(n, result) return result }
In this example, we create a sync.Map called memo to store the results of previous computations. In the fib function, we first check if the result for the current input is already in the memo using the Load method. If it is, we return the result early. Otherwise, we compute the result recursively using the formula fib(n-1) + fib(n-2), store it in the memo using the Store method, and return the result.
Conclusion
In conclusion, both map and sync.Map are useful data structures in Go, but they have different use cases and trade-offs. Maps are fast and simple to use for non-concurrent use, but they require synchronization mechanisms like mutexes for concurrent use. sync.Map provides built-in synchronization and is useful for read-heavy workloads, but it has some limitations and is slower than maps for non-concurrent use. By understanding the differences and trade-offs between these two data structures, we can choose the right one for our specific use case and optimize our code for performance and scalability.
your comments are appreciated and if you wants to see your articles on this platform then please shoot a mail at this address kusingh@programmingeeksclub.com
Thanks for reading 🙂