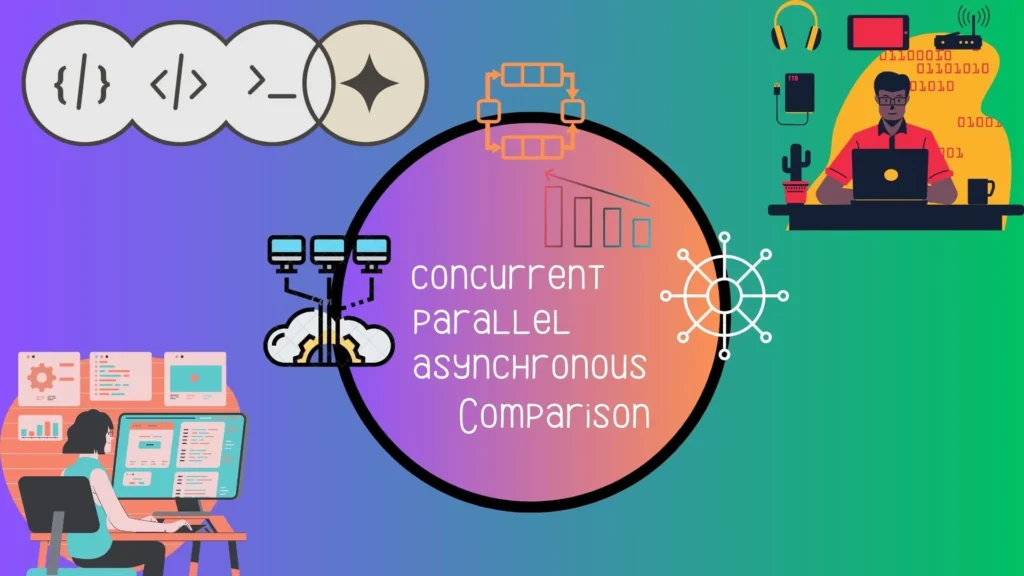
As modern computers have become more powerful and complex, programmers have developed various programming paradigms to improve the efficiency and performance of their programs. Three of the most popular programming paradigms for handling tasks efficiently are parallel, concurrent, and asynchronous programming. Although they share some similarities, there are important differences between them that developers need to understand to choose the right one for the task at hand.
In this blog post, we will explore the differences between them and provide examples to clarify their applications.
Parallel Programming
Parallel programming is a technique for dividing a task into smaller sub-tasks that can be executed concurrently on different processors or cores of a computer. This technique allows programs to take advantage of the parallel processing power of modern computers, which can significantly improve the performance of the program. Parallel programming can be implemented using threads, processes, or GPUs.
In parallel programming, the sub-tasks are independent and do not need to communicate with each other. The main challenge of parallel programming is managing the communication and synchronization between the sub-tasks to avoid conflicts and ensure that the program works correctly. Parallel programming is best suited for tasks that can be easily divided into independent sub-tasks and require a lot of computational power.
Concurrent Programming
Concurrent programming is a technique for executing multiple tasks concurrently and making progress on all of them. Unlike parallel programming, concurrent programming does not require multiple processors or cores. It can be implemented using threads or coroutines.
In concurrent programming, the tasks can be dependent and may need to communicate with each other. The main challenge of concurrent programming is managing the synchronization between the tasks to avoid conflicts and ensure that the program works correctly. Concurrent programming is best suited for tasks that involve I/O operations or user interaction, where the program needs to be responsive and not block the main thread of execution.
Asynchronous Programming
Asynchronous programming is a technique for executing multiple tasks concurrently without blocking the main thread of execution. Asynchronous programming is often used to handle I/O-bound tasks, where the program spends most of its time waiting for I/O operations to complete, such as network communication or disk I/O. Asynchronous programming can be implemented using coroutines or callback functions.
In asynchronous programming, the tasks can be dependent and may need to communicate with each other. However, the communication between the tasks is more complex than in parallel or concurrent programming. The tasks may be executed in a coroutine or callback function, and there is a need for explicit yield points or event loops to switch between tasks. The overhead of managing asynchronous tasks is lower than in parallel or concurrent programming, but the complexity of the programming model can be higher.
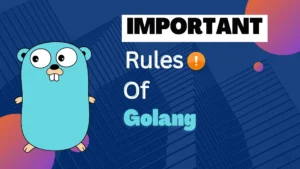
Differences Between Parallel, Concurrent, and Asynchronous Programming
The main difference between parallel programming and concurrent programming is that parallel programming requires multiple processors or cores to execute sub-tasks concurrently, while concurrent programming can execute multiple tasks concurrently on a single processor or core. Another difference is that in parallel programming, the sub-tasks are independent and do not need to communicate with each other, while in concurrent programming, the tasks may be dependent and may need to communicate with each other.
The main difference between asynchronous programming and the other two programming paradigms is that asynchronous programming does not block the main thread of execution while waiting for I/O operations to complete. Asynchronous programming can execute multiple tasks concurrently without the need for multiple processors or cores. Another difference is that in asynchronous programming, the communication between the tasks is more complex than in parallel or concurrent programming, and there is a need for explicit yield points or event loops to switch between tasks.
Working Examples
Let’s take a look at some working examples of parallel, concurrent, and asynchronous programming.
Parallel Programming Example
Here is an example of parallel programming using the Python multiprocessing library:
import multiprocessing def square(number): return number * number pool = multiprocessing.Pool() numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] results = pool.map(square, numbers) print(results)
In this example, the square function is executed concurrently on multiple processors using the multiprocessing library. The Pool object is used to create a pool of worker processes that execute the square function on the input numbers. The map method is used to apply the square function to each number in the numbers list, and the results are returned in the results list.
Concurrent Programming Example
Here is an example of concurrent programming using the Python threading library:
import threading def print_numbers(): for i in range(1, 11): print(i) def print_letters(): for letter in 'abcdefghij': print(letter) t1 = threading.Thread(target=print_numbers) t2 = threading.Thread(target=print_letters) t1.start() t2.start() t1.join() t2.join()
In this example, two tasks are executed concurrently using the Thread class from the threading library. The print_numbers function and the print_letters function are executed in separate threads, allowing both tasks to make progress at the same time. The join method is used to wait for the threads to finish before exiting the program.
Asynchronous Programming Example
Here is an example of asynchronous programming using the Python asyncio library:
import asyncio async def say_hello(): print('Hello') await asyncio.sleep(1) print('World') asyncio.run(say_hello())
In this example, the say_hello function is executed asynchronously using the asyncio library. The async keyword is used to define an asynchronous function, and the await keyword is used to indicate a coroutine that needs to be waited for before continuing. The asyncio.sleep method is used to simulate a long-running task, allowing other coroutines to be executed in the meantime. The asyncio.run method is used to run the coroutine and wait for it to finish before exiting the program.
Best FREE YouTube Video Downloader (2023)
Discover the best free YouTube video downloader websites that let you save and change YouTube videos to MP4, MP3, and…
How to Pickle and Unpickle Objects in Python: A Complete Guide
Learn how to pickle and unpickle objects in Python using the pickle module. Find out the benefits, drawbacks and best…
Ultimate Python Multithreading Guide
Master Python multi-threading with our comprehensive guide. Unlock superior performance and efficiency in your Python applications….
Final Word
Parallel, concurrent, and asynchronous programming are three popular programming paradigms for handling tasks efficiently. Parallel programming is best suited for tasks that can be easily divided into independent sub-tasks and require a lot of computational power.Concurrent programming is best suited for tasks that involve I/O operations or user interaction, where the program needs to be responsive and not block the main thread of execution. Asynchronous programming is best suited for handling I/O-bound tasks, where the program spends most of its time waiting for I/O operations to complete.
Understanding the differences between these programming paradigms is essential for developers to choose the right one for the task at hand. By selecting the right programming paradigm, developers can improve the efficiency and performance of their programs and provide a better user experience for their users.