In this article we are going to implement an approach on how we can reverse an array or string. In this article I’m going to implement for type int but you can also use it for type string as well after doing few modifications in code which is not hard as i understand, I’m going to implement it in two ways first in iterative way and another recursive way so without further ado let’s start implementing it.
Examples:
Input : arr := []int{2,3,4,5,6,7}
Output : arr := []int{7,6,5,4,3,2}
Input : arr := []int{2,4,6,8}
Output : arr := []int{8,6,4,2}
Iterative Way
- Initialize start and end indexes as start = 0, end = n-1
- In a loop, swap arr[start] with arr[end] and change start and end as follows :
start = start +1, end = end – 1
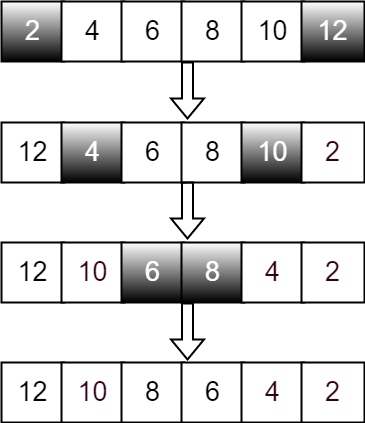
Below is the implementation of the above approach :
package main import ( "fmt" ) // function to reverse given arr without generating new one // with the help of left and right pointers func reverse(start, end int, arr []int) { for start < end { // swapping both indexes // using left and right pointers arr[start], arr[end] = arr[end], arr[start] // moving forward start++ // moving backwards end-- } } func main() { // non-reversed array arr := []int{1,2,3,4,5,6} // left pointer start := 0 // right pointer end := len(arr) - 1 fmt.Printf("non reversed array: %d\n", arr) // calling reverse function reverse(start, end, arr) fmt.Printf("reversed array: %d\n", arr) }
Output:
non reversed array: [1 2 3 4 5 6]
reversed array: [6 5 4 3 2 1]
Time Complexity : O(n)
Recursive Way
- Initialize start and end indexes as start = 0, end = n-1
- Swap arr[start] with arr[end]
- Recursively call reverse for rest of the array.
Below is the implementation of the above approach :
package main import ( "fmt" ) // function to reverse given arr without generating new one // with the help of left and right pointers func recursiveReversed(start,end int, arr []int) { if start>end{ return } arr[start], arr[end] = arr[end],arr[start] recursiveReversed(start+1, end-1,arr) } func main() { // non-reversed array arr := []int{1,2,3,4,5,6} // left pointer start := 0 // right pointer end := len(arr) - 1 fmt.Printf("non reversed array: %d\n", arr) // calling recursiveReversed function recursiveReversed(start, end, arr) fmt.Printf("reversed array: %d\n", arr) }
Output :
non reversed array: [1 2 3 4 5 6]
reversed array: [6 5 4 3 2 1]
Time Complexity : O(n)
That’s all about reversing array in golang