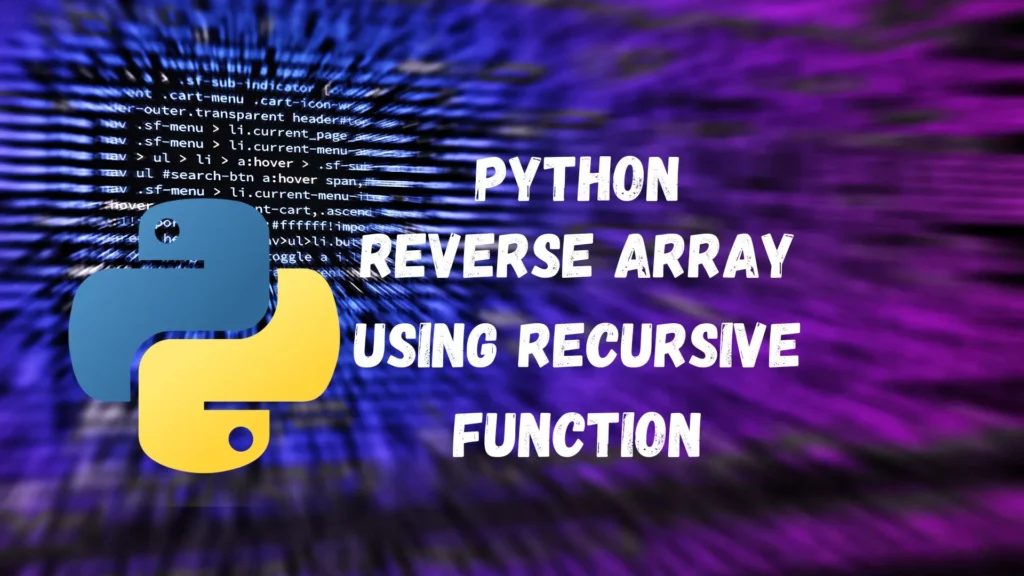
In this article we are going to cover up how we can reverse an array using recursive function, provided code can also be work on string input as well.
What is Recursion?
Recursion is a process in which a function calls itself as a subroutine.
This allows the function to be repeated several times, since it calls itself during its execution. Functions that incorporate recursion are called recursive functions.
Recursion is often seen as an efficient method of programming since it requires the least amount of code to perform the necessary functions. However, recursion must be incorporated carefully, since it can lead to an infinite loop if no condition is met that will terminate the function, so it also have drawbacks.
Program Flow
- we are going to use depth way to get the each value of array from ending to beginning.
- Handling the indexes to don’t get bounce error from it(index not exists kind) via adding if condition.
- directly appending each index to a array variable.
- Begin process again from 1 means calling function to iterate until 2 step not get it wants.
Code Implementation
arr = [10, 23, 12, 1, 2, 65, 89, 8, 5, 90] def recursion(arr, depth): str = [] # step 1 if depth == -1: #step 2 return str str.append(arr[depth]) #step 3 n = recursion(arr, depth - 1) #step 4 if len(n) > 0: str.extend(n) return str print("non recursive arr: ", arr) r = recursion(arr, len(arr) - 1) print("recursive arr: ", arr)
First we have created a array variable called arr which filled with the inputs, then we have a function called recursion which takes two parameters arr and depth.
you know of arr but you might be confused of depth variable, so as you can see in code we are providing depth to recursive function and in recursive we are checking the depth first so we can have our termination condition whenever depth values becomes -1 it will directly return back from that state and as well decreasing the value of depth so we can process to the next index of arr so depth is working like index step 4.
If Step 2 is false then we are appending that arr index value into str array step 3.
In step 4 we are calling recursive function itself so it can process for each index of arr variable.
After that we have condition which is checking n which is a array if length of n > 0 then we are extending the str array with the n array returned by recursive function itself.
non recursive arr: [10, 23, 12, 1, 2, 65, 89, 8, 5, 90]
recursive arr: [10, 23, 12, 1, 2, 65, 89, 8, 5, 90]
your comments are appreciated and if you wants to see your articles on this platform then please shoot a mail at this address kusingh@programmingeeksclub.com
Thanks for reading 🙂