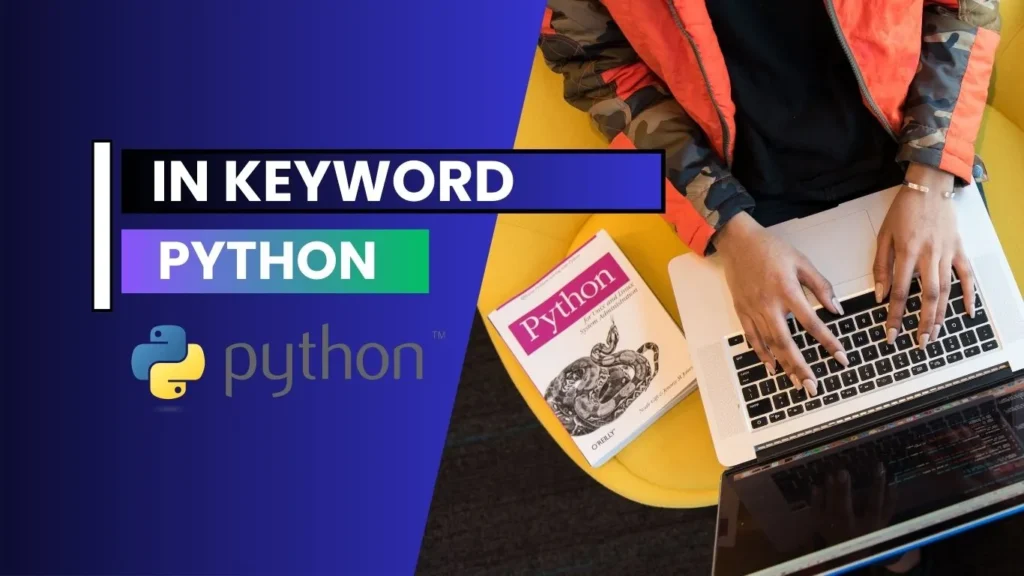
As a part time Python developer, I have found the in keyword to be an incredibly useful tool when working with collections of data. In this article, I will show you the various ways in which the in keyword can be used in Python, and provide some examples of its functionality as well.
What is In Keyword in Python
The in keyword is used to check if an element exists within a collection. The collection can be a list, tuple, set, dictionary, or even a string. The syntax for using the in keyword is simple: element in collection. The in keyword returns a boolean value of True if the element exists within the collection, and False if it’s not.
In Python Keyword in Action
One of the most common use cases of the in keyword is checking if an element exists within a list. For example, consider the following code:
my_list = [1, 2, 3, 4, 5] if 3 in my_list: print("3 exists in the list") else: print("3 does not exist in the list")
In this example, the in keyword is used to check if the value 3 exists within the list my_list. Since 3 does exist within the list, the output of this code will be “3 exists in the list“.
The in keyword can also be used to check if a substring exists within a string. For example:
my_string = "Hello, world!" if "world" in my_string: print("The word 'world' exists in the string") else: print("The word 'world' does not exist in the string")
In this example, the in keyword is used to check if the substring “world” exists within the string my_string. Since “world” does exist within the string, the output of this code will be “The word ‘world’ exists in the string”.
Another interesting use case of the in keyword is checking if a key exists within a dictionary. For example:
my_dict = {"key1": "value1", "key2": "value2"} if "key1" in my_dict: print("The key 'key1' exists in the dictionary") else: print("The key 'key1' does not exist in the dictionary")
In this example, the in keyword is used to check if the key “key1” exists within the dictionary my_dict. Since “key1” does exist within the dictionary, the output of this code will be “The key ‘key1’ exists in the dictionary”.
Finally, it is worth noting that the in keyword can also be used in conjunction with the not keyword to check if an element does not exist within a collection. For example:
my_list = [1, 2, 3, 4, 5] if 6 not in my_list: print("6 does not exist in the list") else: print("6 exists in the list")
In this example, the not keyword is used in conjunction with the in keyword to check if the value 6 does not exist within the list my_list. Since 6
does not exist within the list, the output of this code will be “6 does not exist in the list”.
Conclusion
In conclusion, the in keyword is a powerful tool in Python that can be used to check if an element exists within a collection or not. It can be used with lists, tuples, sets, dictionaries, and strings, and can be negated using the not keyword. I hope this article has been informative and has helped you understand the functionality of in keyword of python.
your comments are appreciated and if you wants to see your articles on this platform then please shoot a mail at this address kusingh@programmingeeksclub.com
Thanks for reading 🙂