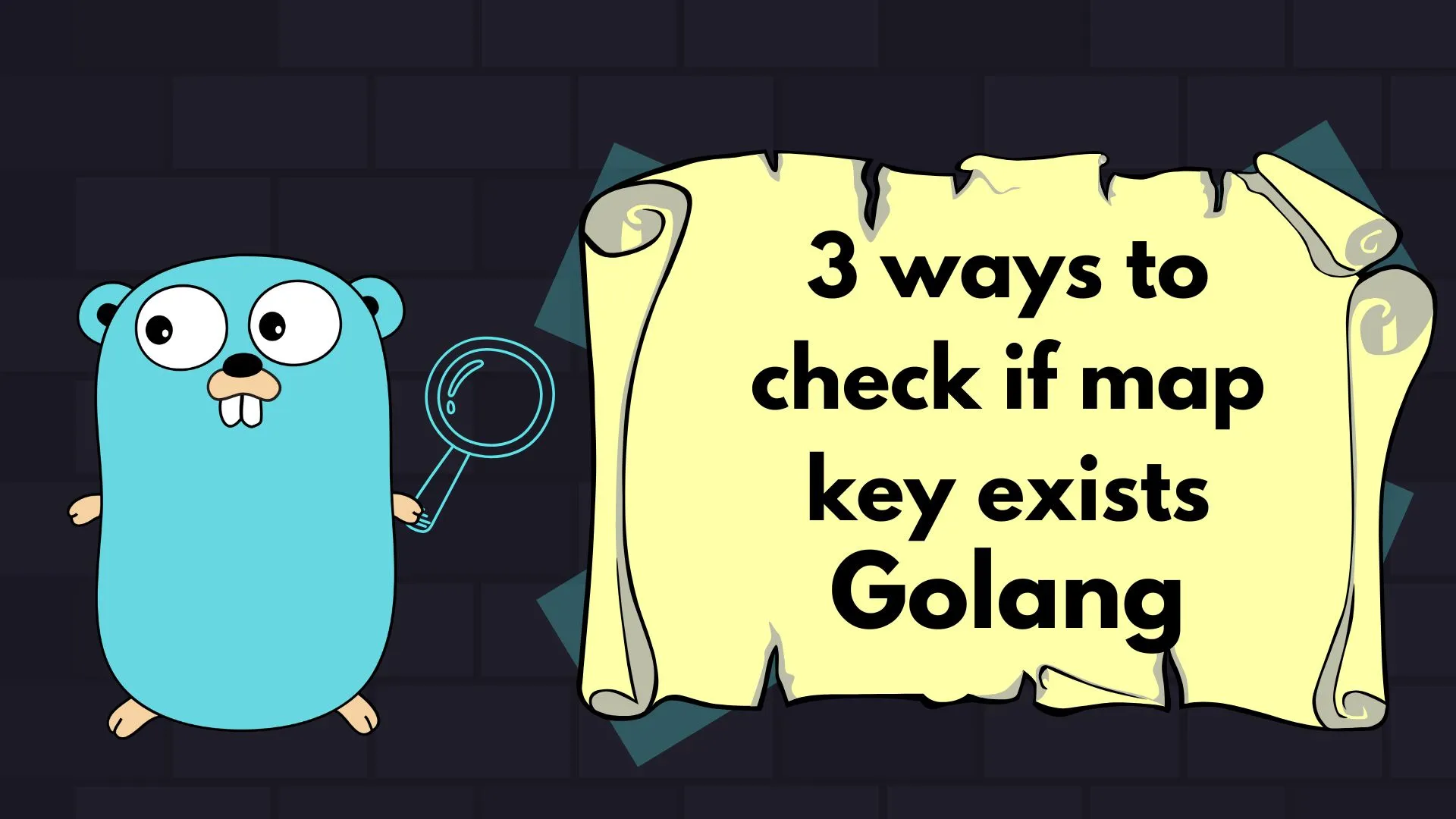
Go map are almost like json, as we access value of json via key we also do the same with map of Go, There are multiple ways to check if a key exists in a map. In this article we are going to learn about how we can check if a key exists in a Go map. There is no fixed number of ways we can check for a key in map it depends on the developer in which way he wants to check the key, basically it depends on the requirements if he require only checking and no need of the value of key then he can use blank identifier for value variable of the key but, we are going to learn below mentioned 3 ways to check if key exists in map or not.
- Using if statement and accessing value of key as well.
- Using if statement and blank value identifier
- Using Go map index notation
Using if statement and accessing value of key as well
The if statement in Go can contain both: condition and a initialization statement. Below is the code for the way one of checking map for key
package main import ( "fmt" ) func main() { m := map[string]int{ "Monday": 1, "Tuesday": 2, "Wednesday": 3, "Thursday": 4, "Friday": 5, "Saturday": 6, "Sunday": 7, } key := "Sunday" fmt.Println("EXAMPLE 1: Using If Statement") //exists will be true if the key contains in m if value, exists := m[key]; exists { //key exists fmt.Printf("map contains the key\nkey = %s\nvalue = %d\n", key, value) } else { //key does not exist fmt.Printf("Map does not contains the key\nkey = %s\n", key) } }
Output:
$ go run main.go
EXAMPLE 1: Using If Statement
map contains the key
key = Sunday
value = 7
So as you can see we have a map called m and a variable called key, In go if you pass a key into the map like this then the map returns two variables first value(whatever the data type you have in your map it will return that) and second is the status which is a boolean type and it returns the true if it founds the passed key in the given map and if not then it will return false, So in the above code we are doing the same but we are doing checking it is recommended way by Go dev but I don’t recommend it because the value is not accessible outside the if block so if you don’t want to make nested conditions then don’t use this approach below is the code if you switch the condition from to false check the below code and it will fail to execute as well
package main import ( "fmt" ) func main() { m := map[string]int{ "Monday": 1, "Tuesday": 2, "Wednesday": 3, "Thursday": 4, "Friday": 5, "Saturday": 6, "Sunday": 7, } key := "Sunday" fmt.Println("EXAMPLE 1: Using If Statement") //exists will be true if the key contains in m if value, exists := m[key]; !exists { //key does not exist fmt.Printf("Map does not contains the key\nkey = %s\n", key) return } //key exists fmt.Printf("map contains the key\nkey = %s\nvalue = %d\n", key, value) }
Output:
$ go run .\main.go
# command-line-arguments
.\main.go:23:5: value declared but not used
.\main.go:29:66: undefined: value
So as you can see for making it execute you now need to declare value variable so it can be access outside the condition.
Using if statement and blank identifier for value
If your purpose is to check only if a map contains key or not and you don’t care about the value of that key, you can use the blank identifier which is ( _ “underscore variable”) in the place of the first return value like mentioned below in the code.
package main import "fmt" func main() { m := map[string]int{ "Monday": 1, "Tuesday": 2, "Wednesday": 3, "Thursday": 4, "Friday": 5, "Saturday": 6, "Sunday": 7, } key := "Sunday" fmt.Println("EXAMPLE 2: If statement and the blank identifier for value") //exists will be true if the key contains in m if _, exists := m[key]; exists { //key exists fmt.Printf("map contains the key\nkey = %s\nvalue = %d\n", key, m[key]) } else { //key does not exist fmt.Printf("Map does not contains the key\nkey = %s\n", key) } }
Using Go map index notation
For this way the steps to check if a map contains a key in using index notation
- Index the map with the given key
- When we index a map in Go, we will get two return values as i mentioned eariler
- First return value contains the value and the second return the value is a boolean that indicates if the key exists or not.
Below is the 3rd and last way to get or check key in a map
package main import "fmt" func main() { m := map[string]int{ "Monday": 1, "Tuesday": 2, "Wednesday": 3, "Thursday": 4, "Friday": 5, "Saturday": 6, "Sunday": 7, } key := "Sunday" fmt.Println("EXAMPLE 3: Using Go Map index notation") //exists will be true if the key contains in m value, exists := m[key] if !exists { //key does not exist fmt.Printf("Map does not contains the key\nkey = %s\n", key) return } //key exists fmt.Printf("map contains the key\nkey = %s\nvalue = %d\n", key, value) }
I recommend this way to check in a map for key and also in this way you can also choose if you want the value or not of the key as well, It’s up to you try to implement that own you own for more understanding about map in Go
Conclusion
We covered the multiple ways of checking if a key exists in a map or not and also which way is better for you as a developer now it also up to you which method you find more understandable and easy to use its up to you.
your comments are appreciated and if you wants to see your articles on this platform then please shoot a mail at this address kusingh@programmingeeksclub.com
Thanks for reading 🙂