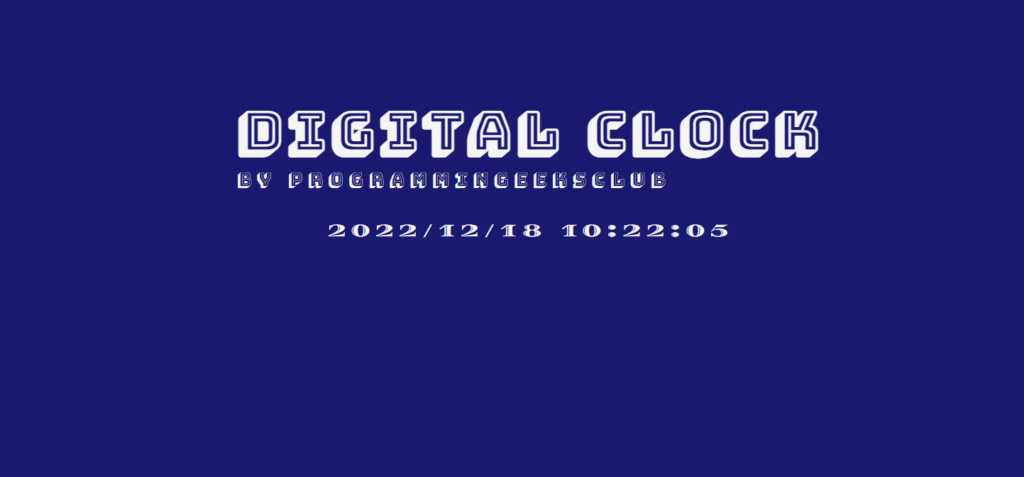
In this tutorial we are going to create a digital clock using HTML, CSS and JavaScript, So how we are going to create a digital clock? Basically we are going to use javascript’s Date() function which will return us the DateConstructor and this DateConstructor have multiple methods, by using those methods we can directly get the each component we need to build our digital clock.
Below is the View of the digital clock we are going to create:
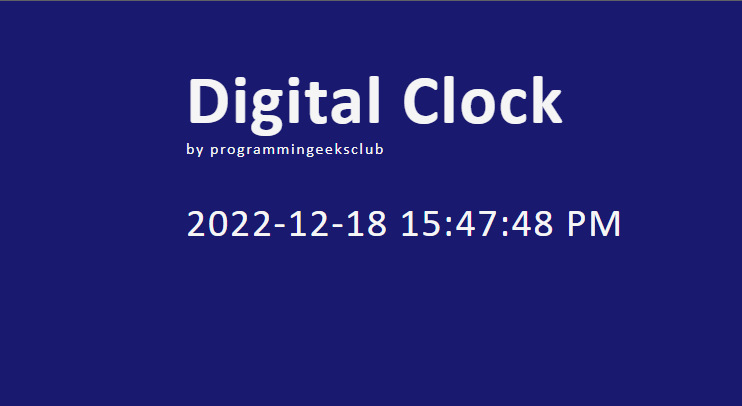
The format of the clock is YYYY/MM/DD HH:MM:SS AM|PM, and our clock is also updating minutes seconds, hours and date accordingly.
To create a digital clock using JavaScript, we can use the setInterval
function to update the time display every 1000 milliseconds (1 second). We will be using the Date
object to get the current time, and then use methods like getFullYear
, getMonth
, getDate
, getHours
, getMinutes
, and getSeconds
to extract the current year, month, date, hour, minute, and second, so as of now we know what we have to do let’s start, but before that I’m going to split the code in three parts: HTML, CSS and JavaScript each one have their own file and in the end we’ll connect CSS and JavaScript with HTML.
Create HTML file
Create a directory called Digital Clock and CD into the Digital Clock directory and open this directory inside any code editor you want.
Once the code editor is loaded create a html file index.html, and paste the following code in that:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Digital Clock Using HTML, CSS and JavaScript</title> </head> <body> <div class="box"> <div> <span> <h1 style="margin-bottom: -30px;">Digital Clock</h1> by programmingeeksclub </span> <p id="digitalClock">2022/12/18 10:22:05</p> </div> </div> </body> </html>
As of now we don’t have any css file yet create if you still try to open this file in browser you’ll see the following output:
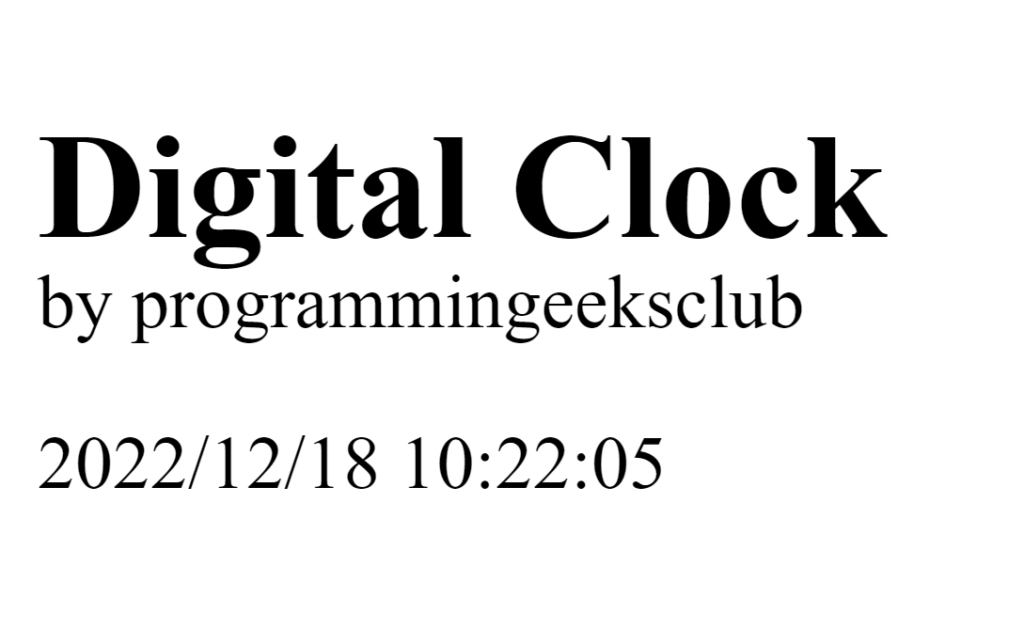
Add Some CSS Design to our Digital Clock
Now let’s add the css into our template to make it look good, for that in the same direcotory let’s create a style.css file and add the following code into it:
@import url('https://fonts.googleapis.com/css2?family=Bungee+Shade&display=swap'); @import url('https://fonts.googleapis.com/css2?family=Diplomata+SC&display=swap'); body { background-color: midnightblue; color: whitesmoke; font-family: 'Bungee Shade', cursive; letter-spacing: 0.1em; } #digitalClock { font-size: 5px; margin-top: -5px; text-align: center; font-family: 'Diplomata SC', cursive; } .box { display: flex; align-items: center; justify-content: center; } h1 { font-size: 15px; } span { font-size: 5px; }
We have our CSS now let’s attach style.css file with our index.html to see the final output, add the following code between head tags of html file.
<link rel="stylesheet" href="./style.css">
Now if you look at the output you’ll see the new look of our digital clock:
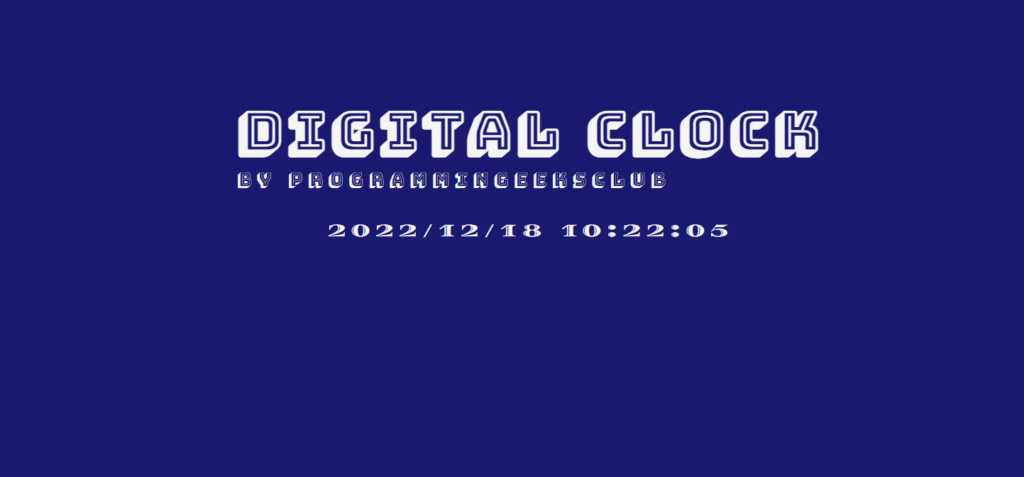
Let’s add JavaScript code to make it funtioning because as of now it’s just a static.
Make our Digital Clock Alive Using JavaScript
Create another file script.js, inside the same directory and connect the file with the html page below is the code to connect your javascript script with html:
Add the following code into your html file before the closing body tag </body>.
<script src="./script.js" async></script>
Now we have connected our script file with html file let’s start writing the script, you can use a live server to check your live code preview, it’s good to work with live preview:
Below is the javascript code:
// make div gloabally accessable var containingItem = document.getElementById('digitalClock'); // give us the zero leading values function ISODateString(d) { function pad(n) { return n < 10 ? '0' + n : n } return d.getFullYear() + '-' + pad(d.getMonth() + 1) + '-' + pad(d.getDate()) + ' ' + pad(d.getHours()) + ':' + pad(d.getMinutes()) + ':' + pad(d.getSeconds()) + ' ' + pad(d.getHours() >= 12 ? 'PM' : 'AM') } let doc = () => { let t = new Date(); containingItem.innerHTML = ISODateString(t); } // set interval 1 sec so our clock // our clock output can update on each // second setInterval(() => { doc() }, 1000);
your comments are appreciated and if you wants to see your articles on this platform then please shoot a mail at this address kusingh@programmingeeksclub.com
Thanks for reading 🙂