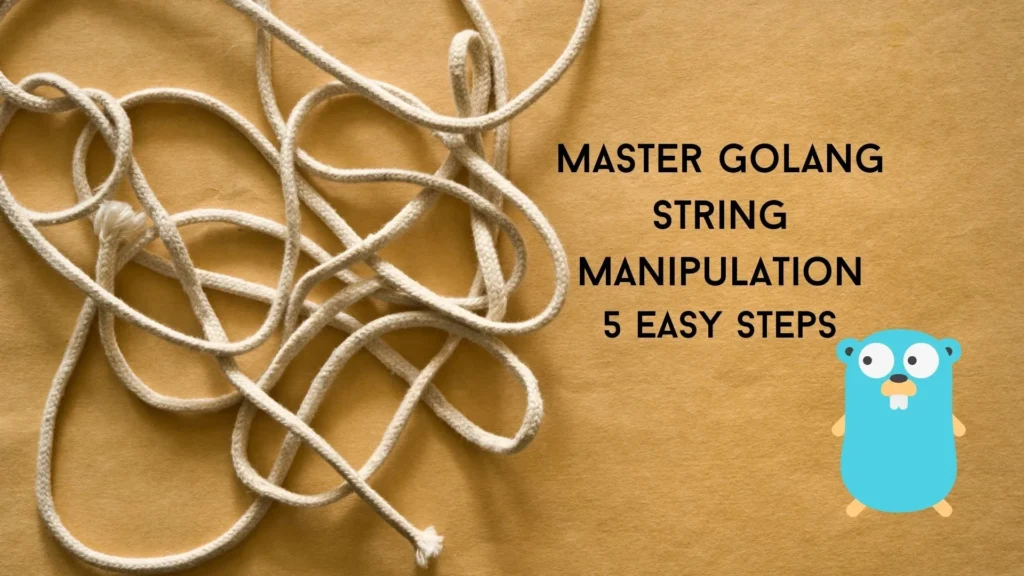
String manipulation is a vital skill for any programmer, and Go(or Golang) offers an elegant and efficient approach to handling strings. In this comprehensive guide, we will delve into five easy steps for mastering Golang string manipulation in Golang.
1. Creating and Initializing Strings
In Golang, strings are immutable sequences of bytes. The bytes are often interpreted as Unicode code points (runes) encoded in UTF-8. Strings are considered values and can be initialized using double quotes or backticks.
Here’s the example of how to create and initialize strings in Golang:
package main func main() { // Using double quotes str1 := "Hello, Golang!" // Using backticks for multi-line strings and preserving whitespaces str2 := `Hello, Golang!` }
In this following code, str1
is initialized with the value “Hello, Golang!” using double quotes. str2
is a multi-line string initialized using backticks, which also preserve whitespaces.
2. Concatenating Strings
In Golang, you can concatenate two strings using the ‘+’ operator. Here’s an example:
package main import ( "fmt" ) func main() { str1 := "Hello" str2 := "Golang" result := str1 + ", " + str2 + "!" fmt.Println(result) // Output: Hello, Golang! }
In this example, we concatenated str1
, a comma, a space, str2
, and an exclamation mark to create the resulting string “Hello, Golang!”, and this is how you can concate the strings in Golang.
For more efficient concatenation, especially with larger strings or multiple concatenations, you can use the strings.Builder
type. This avoids creating multiple temporary strings during concatenation. Let’s see an example:
package main import ( "fmt" "strings" ) func main() { var builder strings.Builder builder.WriteString("Hello") builder.WriteString(", ") builder.WriteString("Golang") builder.WriteString("!") result := builder.String() fmt.Println(result) // Output: Hello, Golang! }
3. Accessing and Modifying Strings
Although strings in Golang are immutable, you can still access individual characters (bytes) by index:
package main import "fmt" func main() { str := "Hello" char := str[1] fmt.Printf("The character at index 1 is: %c\n", char) // Output: The character at index 1 is: e }
In this example, we accessessed the character at index 1 (‘e’) from the string “Hello”.
To modify strings in Golang, you can convert them to a rune slice and then convert the modified rune slice back to a string. example:
package main import "fmt" func main() { str := "Hello" runeSlice := []rune(str) runeSlice[1] = 'a' newStr := string(runeSlice) fmt.Println(newStr) // Output: Hallo }
We created a rune slice from the string “Hello”, modified the rune at index 1 to ‘a’, and then converted the modified rune slice back to a string, resulting in “Hallo”.
4. Working with String Functions
Golang’s strings
package offers a plethora of useful functions for string manipulation. Let’s explore some examples:
package main import ( "fmt" "strings" ) func main() { // To uppercase and lowercase str := "Golang" fmt.Println(strings.ToUpper(str)) // Output: GOLANG fmt.Println(strings.ToLower(str)) // Output: golang // Replace all occurrences of a substring str = "Hello, Golang!" fmt.Println(strings.ReplaceAll(str, "o", "0")) // Output: Hell0, G0lang! // Split a string into a slice of substrings str = "Go,string,manipulation" parts := strings.Split(str, ",") fmt.Println(parts) // Output: [Go string manipulation] str = " Golang is awesome! " trimmedStr := strings.TrimSpace(str) fmt.Printf("[%s]\n", trimmedStr) // Output: [Golang is awesome!] str = "!!Golang!!" trimmedStr = strings.Trim(str, "!") fmt.Printf("[%s]\n", trimmedStr) // Output: [Golang] }
In this example, I showcased various string manipulation functions provided by the `strings` package. We converted a string to uppercase and lowercase, replaced all occurrences of a substring, splitted a string into a slice of substrings, We trimmed whitespaces and exclamation marks from the beginning and the end of strings using the strings.TrimSpace
and strings.Trim
functions as well.
5. Formatting Strings
Golang provides the fmt
package for formatting strings with placeholders. You can use functions like Sprintf
or Printf
to format strings with different types of placeholders.
The following example demonstrates how to format a string using the Sprintf
function:
package main import ( "fmt" ) func main() { name := "Gopher" age := 14 formattedStr := fmt.Sprintf("My name is %s, and I am %d years old.", name, age) fmt.Println(formattedStr) // Output: My name is Gopher, and I am 10 years old. }
In this example, we used the %s
and %d
placeholders to format a string with a string and an integer value. The Sprintf
function returns a new formatted string, which we then used to print using fmt.Println
function.
These are the 5 string manipulation steps using these you can easily manipulate any string as you want.
Conclusion
In this comprehensive guide, we have explored five easy steps to master Golang string manipulation. We have covered creating and initializing strings, concatenating strings, accessing and modifying strings, working with string functions, and formatting strings. With these steps, you are well on your way to becoming a Golang string manipulation expert. Happy coding!